Python ek object oriented programming language hai, iska mtlb ye hai ki Python mai hr ek chiz object hai. Dekho Oops kafi confusing ho skta hai agar ap longo ko oops kafi gande tarike se padhya gya hai , mai is post ke jariye ap longo ko oops ko bahut assan shabdo mai samjhane ki pori koshish krunga.
Aayiye oops padhne se pehle kuch terms clear kr lete hai , taki ap longo ko ise padhne mai koi muskil na ho.
Class and object
Class ek design ya blueprint hai. Object ek real thing hai.
Aayiye ise example ki madad se aur clear krte hai jaise Animal ek class hai aur cat , dog , tiger etc ye sb object hai, Animal ek real chiz nhi hai lekin object ek real chiz hai
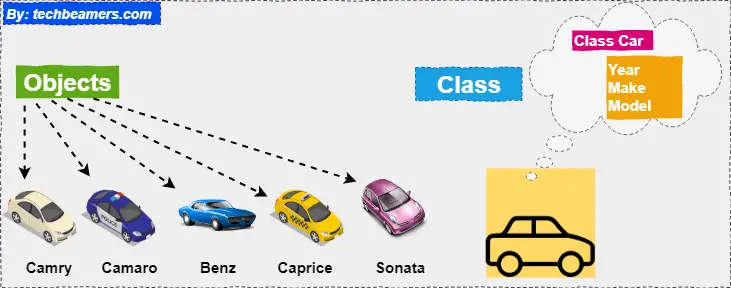
Ek example aur lete hai jaise bhot longo ke pass iPhone 11 hoga lekin ye design sirf ek baar hi kiya jata hai to ek class ke pass kai object ho skte hai.
Aayiye object ko aur deep se samjhte hai
Jaise...
mylist = [ 3,5,6,7]
to hm yaha kahenge ki mylist ek object hai class list ka , lekin ye jo list class hai ye built in class hai, hm yaha khud ki class bana skte hai jise hm user-defined class kahenge.
Object kya hai ?
Object ek real chiz hai, object ke pass attribute aur behaviour hota hai, attribute is like how we look , what we wear ,basically ise variables kahte hai behaviour hamare action ko define krta hai like walking , talking , sleeping etc. ise hm methods kah skte hai.
Function inside a class is called method
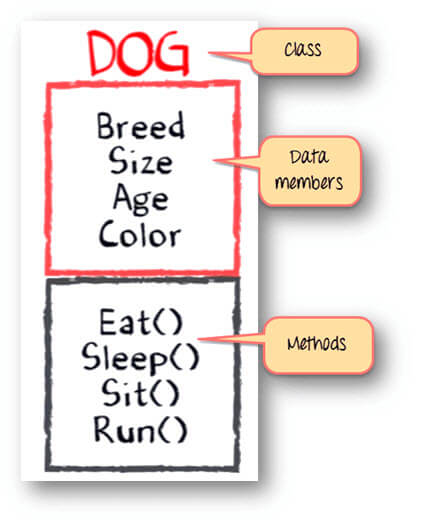
User defined class
Class ko create krne ke liye hme class keyword ka use krna padta hai
Example:
---------------------------------------------------------------------------------
#creating a class
class Computer:
def config(self):
print("i5 , 16gb , 1TB")
#creating an object
com1= Computer()
#Calling an object
Computer.config(com1)
Ouptut:
i5 , 16gb , 1TB
#class ko hm create krte hai class keyword ki madad se
#config yaha ek method hai
#com1 name ka hmne ek object create kiya hai
#Aur fir hmne ise call kiya hai , call krte samay hm kr kya rahe hai ki hmne self(argument) mai com1 name ka parameter pass kiya hai
#Self keyword ki madad se hm kisi bhi python class ke attributes aur method ko acess kr skte hai
#It is good programming practice to start the class name with capital letter
#Another way of calling an object
com1.config()
Output:
i5,16gb 1tb
-------------------------------------------------------------
# Another way of doing this same program
class computer:
def config(self):
print("i5,16gb 1tb")
com1 = computer()
com1.config()
Output:
i5,16gb 1tb
------------------------------------------------------------------------------------
__init__ Method
init stand for intialization , init method ka use hota hai variable ko intialize krne ke liye , init method ki speciality ye hai ki ise hme call nhi krna padta hai ye automatically call ho jati hai object create krte samay.
Let's take an example
------------------------------------------------------------
class grow:
def __init__(self):
print("in init")
def config(self):
print("hello")
com1 = grow()
com1.config()
Output:
in init hello#To ap dekh skte hai ki init method ko hame call nhi krna padta , ye automatically call ho jati hai object create krte samay, ye java ke constructor se kafi similar hai.-----------------------------------------------------------class Computer: def __init__(self, cpu, ram): self.cpu = cpu #intializing the value self.ram = ram def config(self): print(self.cpu , self.ram)
com1 = Computer('i5' ,16) com2 = Computer('Ryzen 3' ,8) com1.config() com2.config()
#Output:
i5 16 Ryzen 3 8# Self parameter basically hr method ka first parameter hota hai, Self name jruri nhi hai hm koi bhi name use kr skte hai lekin most commanly self use hota hai becoz it increase the code readability#Self ka use basically variable ko access krne krne ke liye hota hai jo ki us particular class ko belong krte hai.----------------------------------------------------------class BadmintonPlayer(): def __init__(self,aName,aRacket,aShuttle): print('I m in Constr...') self.name = aName self.racket = aRacket self.shuttle = aShuttle
sindhu = BadmintonPlayer('Sindhu','Yonex','Yonex')
Output:
I m in Constr...--------------------------------------------------------------------------------------class Person(): def __init__(self,aName): print('I m in Constructor') self.name = aName def eat(self): print('Idly') def play(self): print('Badminton') print('or Cricket') def exercise(self): print('Jogging') p1 = Person('Sai') p1.eat() p1.play() p1.exercise() p1.name
Output:
Comments
Post a Comment