A data structure is a collection of different forms and different types of data that has a set of specific operations that can be performed
Python mai data structure two categories mai divided hai pahla hai built in data structure (built in ka mtlb hai jo ki python ke undar hi hai hame inhe bahar se import krke lane ki koi jrurt nhi hai, aur dusra hai user defined data structure , is post mai hm python ke built in data structure ki baat krenge.
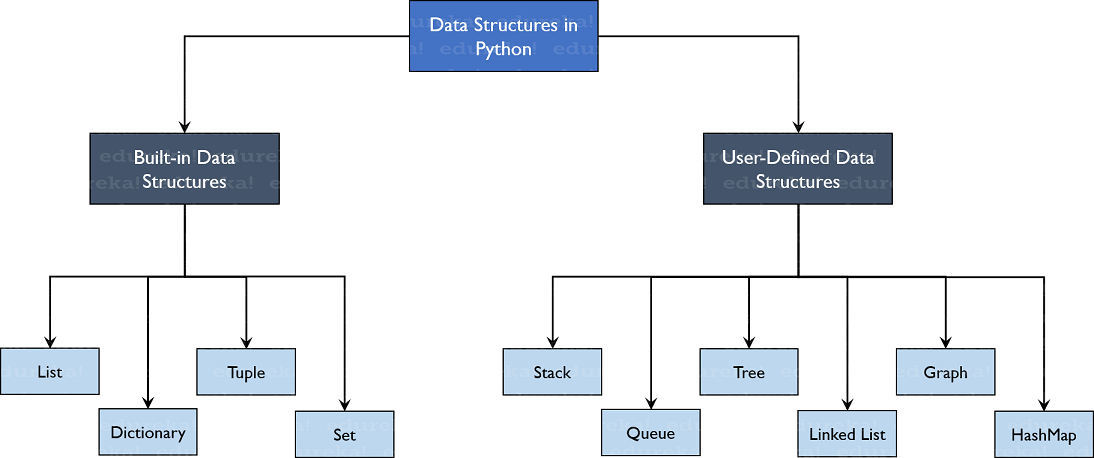
Built-in Data Structure
- Lists
- Dictionary
- Tuple
- Set
1.Lists
List basically ek container hai jisme hm bahut saare object rakh skte hai , List mai hm textual data aur numerical data dono rakh skte hai, chaliye ise aur clear banate hai ek example ki madad se , es example ko samajhne ke liye apko kuch assumption krne padenge jaise ki hm numerical data ko tea cup manenge
aur textual data ko coffee cup
numerical data - tea cup
textual data - coffee cup
to is hisab se list ho gyi tray of coffee cup and tea cup , to basically us tray ko hm list bol rahe hai.
Aur is list mai hm object nikal skte hai , object jod skte hai, yani ham us tray me aur coffee ya tea cup jod skte hai aur us tray se ham coffee ya tea cup nikal bhi skte hai.
"list mai jitne chahe utne tea cup , coffee cup ho skte hai aur nhi bhi ho skte hai"
" List ko square bracket ([]) mai likha jata hai"
chaliye ise aur clear banate hai...........
Syntax of list
L = []
Examples
-------------------------------------------------------------------------------------------------------------
grocery = ["harpic", "vim bar", "deodrant","bhindi","lollypop", 56]
print(grocery)
['harpic', 'vim bar', 'deodrant', 'bhindi', 'lollypop', 56]
# basically hamne grocery naam ki ek list banayi hai jisme numerical data aur textual data dono hai
----------------------------------------------------------------------------
print(type(grocery))
<class 'list'>
#ye hame bata rha hai ki grocery ek list type data structure hai
------------------------------------------------------------------------------
print(grocery[1])
print(grocery[0])
print(grocery[5])
vim bar
harpic
56
#yaha hm list se value nikal rahe hai index number daalke , jaisa ki hm jante hai python follow zero based index system
yani python mai counting zero se start hoti hai , to index number 1 mai vimbar hai, index number 2 mai harpic hai
index number 5 mai 56 hai
------------------------------------------------------------------------------------
grocery.append(8)
print(grocery)['harpic', 'vim bar', 'deodrant', 'bhindi', 'lollypop', 56, 8]
#append mtlb end mai jod do , isliye hamne list mai sbse end mai 8 ko jod diya
#pr agar hame end mai nhi kisi dusri position mai koi value insert krni hai to kaise kr skte hai aayi dekhte hai
--------------------------------------------------------------------------------------
grocery.insert(2 , "rajma")
print(grocery)['harpic', 'vim bar', 'rajma', 'deodrant', 'bhindi', 'lollypop', 56, 8]
#isme hm 2nd postion mai "rajma" jod rahe hai#agar hme list se koi value nikalni hai to hm kya krenge aayie dekhte hai-----------------------------------------------------------------------------------grocery.remove("rajma")
print(grocery)
['harpic', 'vim bar', 'deodrant', 'bhindi', 'lollypop', 56, 8]
#remove function as a name suggest ye remove krte hai chijo ko
---------------------------------------------------------------------------------
" Ek chiz mai apko bta ki list ek class hai aur us class ke undar append , insert , remove ye sb
list class ke function hai"
------------------------------------------------------------------------------------------
numbers=[2,4,5,11,9]
print(numbers)[2, 4, 5, 11, 9]
#Yaha hamne ek aur list li hai jiska naam numbers hai
----------------------------------------------------------------
numbers.sort()
print(numbers)[2, 4, 5, 9, 11]
#yaha hamne sorting ki hai list mai sort function ka use krke , python mai sorting by default asscending order mai hoti hai
--------------------------------------------------------------------------------------
print(numbers[:])
[2, 4, 5, 9, 11]
#ye ek aur tarika hai list ko access krne ka
---------------------------------------------------------------------------------------------
print(numbers[1:])[4, 5, 9, 11]#basically hm bol rahe hai ki index no. 1 se lekar jitni bhi value hai list mai use print kra do----------------------------------------------------------------------------------print(numbers[1:4])[4, 5, 9 ]#yaha hm bol rahe hai ki hame index no. 1 se lekar index number 4 tak value do#pr yad rahe [1:4] mai 1 included rahega aur 4 excluded rahega yani hame sirf third position tak value milegi-------------------------------------------------------------------------print(len(numbers))5# ham dekh skte ki list mai 5 value hai , isliye is list ki length 5 aayi hai---------------------------------------------------------------------------------print(max(numbers))
print(min(numbers))112# ye list ka max aur min number bata raha hai-------------------------------------------------------------------------------------2.TupleTuple list jaisa hi hai bs list aur tuple mai thoda difference hai list mutable hoti mtlb hmlist ko change kr skte hai lekin Tuple immutable hota hai yani tuple ko change nhi karaya ja sktaTuple ko round bracket () ke andar likha jata haiSyntax :t =()Examples:---------------------------------------------------------------
tp=(1,2,6,7)
print(tp)
(1, 2, 6, 7)
#tp ek tuple hai
---------------------------------------------------------------------------------
thistuple = ("apple", "banana", "cherry")
print(thistuple)
('apple' , 'banana' , ' cherry' )
#ye ek aur tuple hai
------------------------------------------------------------------------------
print(thistuple[1])
banana
#isme hm list ki tarah value nikal skte ha
------------------------------------------------------------
3. Dictionary
Dictionary is a key value pair , agar saral shabdo mai kahe to python dictionary mai key hoti hai aur ussse associated value hoti hai. Dictionary sbse jada flexible built- in datatype hai python mai.
List mai hame agar koi value nikalni ya insert krna hai to hame index number dena padta tha , lekin dictionary mai hame agar koi value nikalni hai ya insert krni hai to hame key dena padta hai
Dictionary is nothing but key value pair
Python dictionary ko curly bracket se represent kiya jata hai
syntax
dict ={}
---------------------------------------------------------
dict = {'Coffee': 'Nescafe', 'Taj':'Tea', 'Tulasi':'Gree Tea'}
dict
{'Coffee': 'Nescafe', 'Taj': 'Tea', 'Tulasi': 'Gree Tea'}
#yaha hmne dictionary print krayi hai
# ap dekh skt hai ki coffee key hai aur Nescafe value hai, isi tarah taj key hai aur tea value hai
--------------------------------------------------------
dict['Coffee']
'Nescafe'# hmne key dala to hame value mil gyi# lekin list mai aisa nhi hota---------------------------------------------------------wtr1 = ['Coffee', 'Tea', 'Green Tea'] wtr1[0]
'Coffee'#list mai hame index number daalna padta hai kisi value ko nikalne ke liye----------------------------------------------------------------------# another dictionarywtr2 = {'Coffee': 'Nescafe', 'Brook Bond':'Tea', 'Taj':'Green Tea'} wtr2
{'Coffee': 'Nescafe', 'Brook Bond': 'Tea', 'Taj': 'Green Tea'}------------------------------------------------------------------------------------wtr2['Coffee'] = 'Bru' wtr2
{'Coffee': 'Bru', 'Brook Bond': 'Tea', 'Taj': 'Green Tea'}#ap dekh skte ho ki hmne coffee ko nescafe se bru badal diya----------------------------------------------------------------------------------wtr2['Coffee2'] = 'Frappes' wtr2
{'Coffee': 'Bru', 'Brook Bond': 'Tea', 'Taj': 'Green Tea', 'Coffee2': 'Frappes'}#adding the key and value into the dictionary-----------------------------------------------------------------------------------del wtr2['Coffee2']
wtr2
{'Coffee': 'Bru', 'Brook Bond': 'Tea', 'Taj': 'Green Tea' }#deleting an item in dictionary by using del keyword---------------------------------------------------------------------------------wtr2.keys()
dict_keys(['Coffee', 'Brook Bond', 'Taj' ])#it give all the key in the dictionary wtr2--------------------------------------------------------------wtr2.values()
dict_values(['Bru', 'Tea', 'Green Tea'])#it give all the value in the dictionary wtr2-------------------------------------------------------------------------------4. SetsSets basically unordered collection hota hai unique aur immutable object ka, more precisely set aur list mai ye difference hota hai ki sets mai ham sirf unique element daal skte hai lekin list mai hm duplicate element bhi daal skte hai.Python mai sets ko hm curly braces ke andar likhte hai----------------------------------------------------------------------------d = ({1,2,3,4})
print(d)output:{1, 2, 3, 4}
#yaha hamne d naam ka ek set create kiya hai
----------------------------------------------------------------------------------
print(type(d))output:<class 'set'>
-----------------------------------------------------------------------------------------------------
s = set()
print(type(s))output:<class 'set'>
#yaha hamne s naam ka ek khali set create kiya hai , ye set create krne ek aur tarika hai
--------------------------------------------------------------------
s.add(1)
s.add(1)
s.add(2)
print(s)output:{1, 2}
#jaisa ki maine btaya tha ki set mai hm sirf unique element hi add kr skte hai, to ap yahadekh skte hai ki hamne yaha 1 ko do baar add kiya hai lekin set mai ek hi baar aaya hai kyokiset mai duplicate allowed nhi hai.Agar apne math mai sets ko kahi padha hai , to apko pta hoga hm sets maikafi saare opreation kr skte hai jaise union , intersection, complement aurbhi bahut kuch... , to aayiye kuch example ki madad se hm dekhte hai ki python ki madad se hm sets mai kaise operation kr skte hai----------------------------------------------------------------------------s1 =s.union({3,4,5,6})
print(s , s1)output:{1, 2} {1, 2, 3, 4, 5, 6}
#do sets ke element ko jodne ko hm union kahte hai , aur wahi hamne yaha kiya union keyword ki ma
madad se---------------------------------------------------------------p =({12,13,14,15,16})
f = ({15,16,17})
d = p.intersection(f)
print(d)output:{16, 15}
#intersection mtlb common p aur f set mai 16 aur 15 element aise hai jo ki common hai---------------------------------------------------------------------Sets mai hm aur bhi function ka use kr skte hai unki list ye rahi
Method | Description |
---|---|
add() | Adds an element to the set |
clear() | Removes all the elements from the set |
copy() | Returns a copy of the set |
difference() | Returns a set containing the difference between two or more sets |
difference_update() | Removes the items in this set that are also included in another, specified set |
discard() | Remove the specified item |
intersection() | Returns a set, that is the intersection of two other sets |
intersection_update() | Removes the items in this set that are not present in other, specified set(s) |
isdisjoint() | Returns whether two sets have a intersection or not |
issubset() | Returns whether another set contains this set or not |
issuperset() | Returns whether this set contains another set or not |
pop() | Removes an element from the set |
remove() | Removes the specified element |
symmetric_difference() | Returns a set with the symmetric differences of two sets |
symmetric_difference_update() | inserts the symmetric differences from this set and another |
union() | Return a set containing the union of sets |
update() | Update the set with the union of this set and others |
DOUBT ?
ASK ME ON COMMENT SECTION
Comments
Post a Comment